People just getting started with maven always ask me the same 3 questions, so here’s a a short FAQ!
How to change the output directory?
How to shade dependencies and what it means
Sometimes you are using certain libraries (for example, my CustomBlockData class, or similar stuff) that is not already present at runtime. In those case, you have to shade (and should relocate) those libraries into your own .jar. This basically means that maven will take the dependencies you are using, and also puts them into your .jar file. To achieve this, add the maven-shade-plugin to your pom.xml to <build><plugins>:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <version>3.5.0</version> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> </execution> </executions> </plugin>
Now, it’s very important to properly declare the correct scopes for your dependencies. Every dependency that you do not want to be shaded (Spigot/Bukkit itself, WorldGuard, …) has to be declared using <scope>provided</scope>, while you must use <scope>compile</scope> for the libraries you want maven to actually shade into your .jar file.
Provided means that your plugin expects those classes to already be loaded at runtime, e.g. if it’s Spigot itself, or another plugin. Everything else must be shaded into your plugin.
For example, imagine we are using spigot-api and my CustomBlockData library, you want to set spigot-api to provided and CustomBlockData to compile:
<dependencies> <dependency> <groupId>org.spigotmc</groupId> <artifactId>spigot-api</artifactId> <version>1.18.2-R0.1-SNAPSHOT</version> <scope>provided</scope> </dependency> <dependency> <groupId>com.jeff_media</groupId> <artifactId>CustomBlockData</artifactId> <version>1.0.5</version> <scope>compile</scope> </dependency> </dependencies>
How to change your Java version
You can easily change the targeted java version using maven. To do so, add the maven-compiler-plugin if you don’t already have it declared into your <build><plugins> section, or change the existing declaration. For example, for Java 17, you can use this:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>17</source> <target>17</target> </configuration> </plugin>
Important: You must also tell your IDE to use at least the specified Java version to run maven in the first place. In IntelliJ, you can click on File -> Project Structure -> Project Settings -> Project, and set the “SDK” to a java version that’s equal or higher to your targeted Java version.
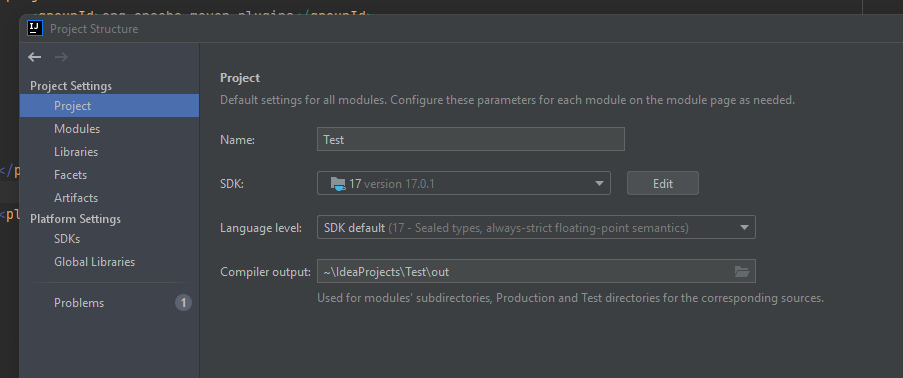