Spigot uses Log4j to log console output, hence making it easy to listen to incoming console messages, or to block certain console messages from appearing.
Prequisities
You need to have Log4J on your classpath. We simply add the dependency to our pom.xml
using the provided
scope, as Spigot already contains those classes on runtime:
<dependency> <groupId>org.apache.logging.log4j</groupId> <artifactId>log4j-core</artifactId> <version>2.19.0</version> <!-- This is the version Spigot 1.20.1 uses --> <scope>provided</scope> </dependency>
Create a custom Log Filter
Now we create a custom log filter by extending Log4J’s AbstractFilter
that overrides the filter(LogEvent)
method. In this example, we simply forward all console messages to all players. As it’s important that you are using the correct imports (usually org.apache.logging.log4j.core.*
), I have included them in the following code examples:
import org.apache.logging.log4j.core.LogEvent; import org.apache.logging.log4j.core.filter.AbstractFilter; import org.bukkit.Bukkit; import org.bukkit.ChatColor; import org.bukkit.entity.Player; public class MyLogFilter extends AbstractFilter { @Override public Result filter(LogEvent event) { String message = event.getMessage().getFormattedMessage(); String sender = event.getLoggerName(); for(Player player : Bukkit.getOnlinePlayers()) { player.sendMessage("", ChatColor.GOLD + "[" + sender + "]", ChatColor.RESET + message); } return Result.NEUTRAL; } }
There’s a few things I gotta explain about that:
- We do not use
Bukkit.broadcastMessage(String)
to forward the log messages to all players, becausebroadcastMessage(String)
itself gets logged to console, so we’d end up with infinite recursion. - The sender is the name of the logger, which will usually be the class name that created this logger.
- At the end, we return
Result.NEUTRAL
. If we’d returnResult.ACCEPT
, this message would be sent to console, no matter whether other filters would block this message. If we’d returnResult.DENY
, this message would not be sent to console. UsingResult.NEUTRAL
however, we simply don’t change the state of this message, so the console behaves exactly as if we were not listening to it.
Attach the custom Log Filter
Now all we gotta do is to instantiate our custom Filter and attach it to the root logger. Again, it’s very important that you are using the correct imports!
import org.apache.logging.log4j.LogManager; import org.apache.logging.log4j.core.Logger; import org.apache.logging.log4j.core.Filter; import org.bukkit.plugin.java.JavaPlugin; public class ServerDebug extends JavaPlugin { @Override public void onEnable() { Logger rootLogger = (Logger) LogManager.getRootLogger(); Filter myFilter = new MyLogFilter(); myFilter.start(); rootLogger.addFilter(myFilter); } }
Let’s try it out
That’s basically everything. Let’s try it out – I wrote a tiny command using ACF that just outputs two messages to console, once using the plugin’s logger, and once using the global Bukkit logger:
@CommandAlias("serverdebug") public class DebugCommand extends BaseCommand { private final ServerDebug plugin; public DebugCommand(ServerDebug plugin) { this.plugin = plugin; } @Default public void onOutput(CommandSender sender) { plugin.getLogger().info("This is a message printed by a plugin using plugin.getLogger().info(\"...\")"); Bukkit.getLogger().info("This is message printed by a plugin using Bukkit.getLogger().info(\"...\")"); } }
Here’s the console output:

And here you can see our filter properly forwarding all the console messages:
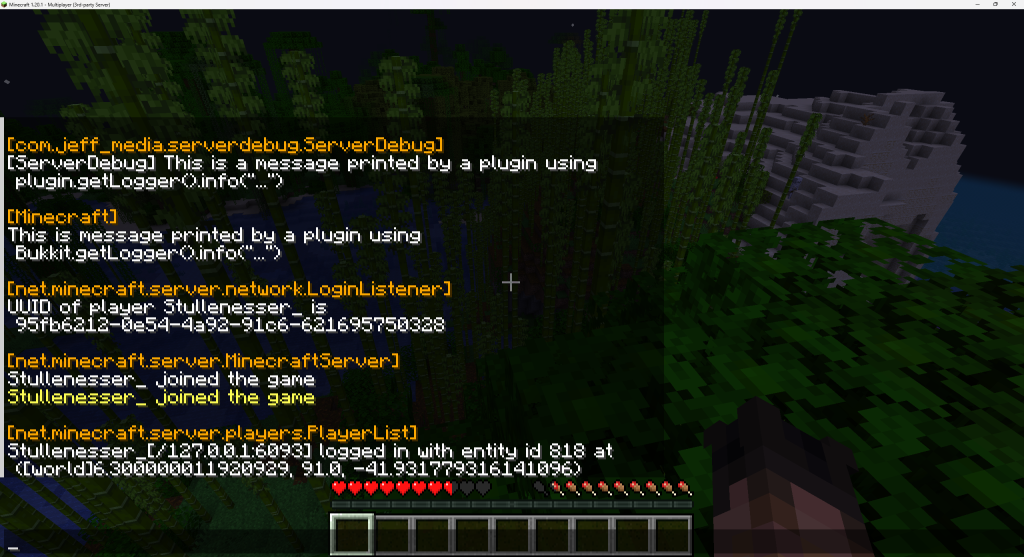
As you see, I also joined the game with my alt account, and now we can notice that the vanilla game uses three different loggers when a player logs in:
- LoginListener to print the UUID
- MinecraftServer to send the actual join message (which here appears twice, once because it got logged to console, and the colored message is the actual in-game join message)
- PlayerList, which informs us about the IP address, entity ID, and location
How to filter (“block”) certain console messages
As mentioned earlier, we can use the return value in Filter#filter(LogEvent)
to filter out unwanted messages. For example, let’s filter out all messages that contain the String mfnalex
:
public class MyLogFilter extends AbstractFilter { @Override public Result filter(LogEvent event) { String message = event.getMessage() .getFormattedMessage(); if(message.contains("mfnalex")) { return Result.DENY; // mfnalex is a ninja } return Result.NEUTRAL; } }
Quickly using the /say
command from console confirms that it’s working as intended:

Issues when using Paper?
If you’re running Paper instead of Spigot, you’ll notice a ton of weird symbols in the console because of the color codes Paper adds to the console output. For example, let’s enter the /version
command in console:

The actual message that gets passed to our filter also contains all those nasty color codes:
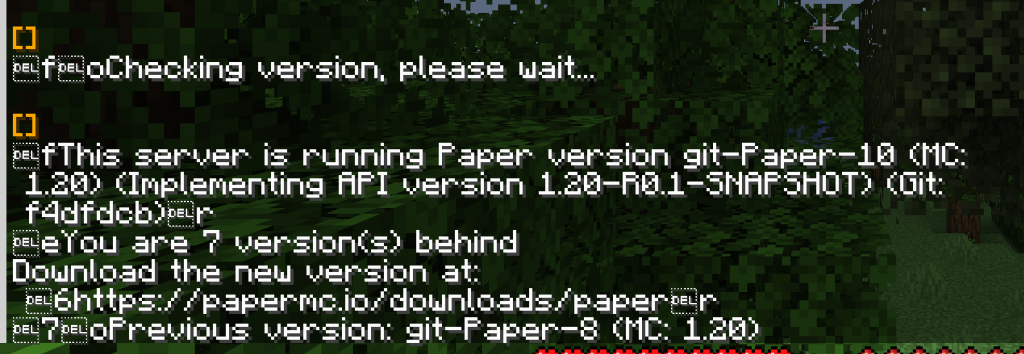
I don’t know if there’s an easy way to filter those out, or make Paper stop adding distracting colors into the console output. So, if you do use Paper, you’ll have to figure out yourself how to prevent this.