If you’re using maven for your Spigot plugins (which you should do), it’s easy to make maven automatically save your plugin’s .jar in your plugins folder.
There’s two ways of doing this:
1. The lazy way (not recommended)
If you only work alone on one computer, you can just directly declare the output location in the “maven-jar-plugin”.
You can simply add a <outputDirectory> tag to your maven-jar-plugin. If you already have this plugin declared, add it to the existing configuration. Otherwise, just add it inside <project><build><plugins>, like this:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <version>3.3.0</version> <configuration> <outputDirectory>C:\MyTestServer\plugins\</outputDirectory> </configuration> </plugin>
It should look like this:
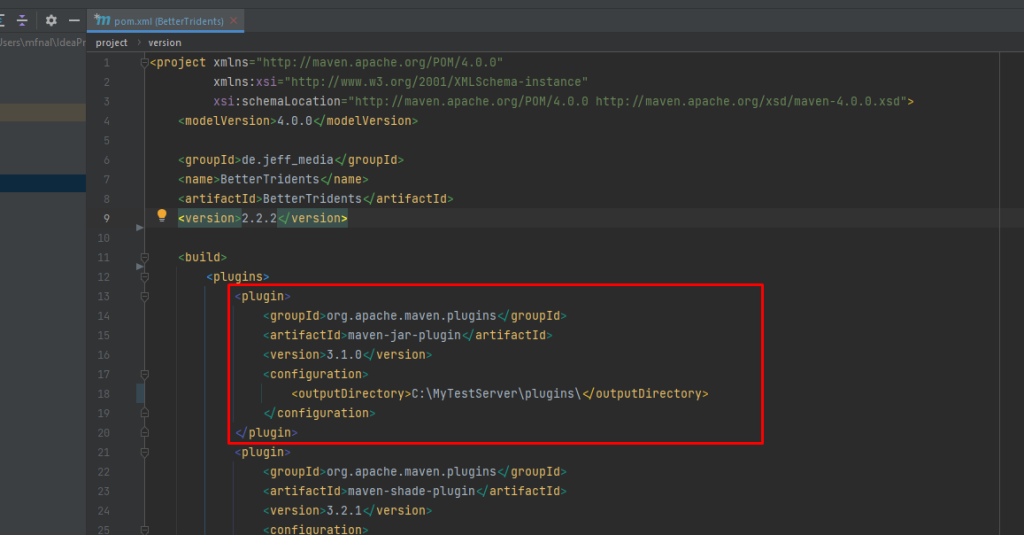
If you want to also change the file name itself, you can add <finalName>MyPlugin</finalName>
to the configuration – now your jar gets saved as MyPlugin.jar. The default value for that is ${project.name}-${project.version}
by the way.
2. The proper way: using maven profiles
If you work together with other people, a better solution would be to include a profile in your pom, so that your build won’t fail or put files into random destinations for other people.
To do this, we just declare a new profile in your <project><profiles> section, and then change the maven-jar-plugin’s configuration there:
<profiles> <profile> <id>export-to-test-server</id> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <configuration> <outputDirectory>/Users/mfnalex/mctest/plugins</outputDirectory> </configuration> </plugin> </plugins> </build> </profile> </profiles>
Please note that if you’re declaring the output directory in the profile, you should not declare it anywhere else. So if you used the “lazy way” before (as described above), get rid of your existing <outputDirectory> part from your global <build><plugins> section.
Of course, you can again change the file name itself using <finalName>
in the configuration.
Your final pom now should look like this:
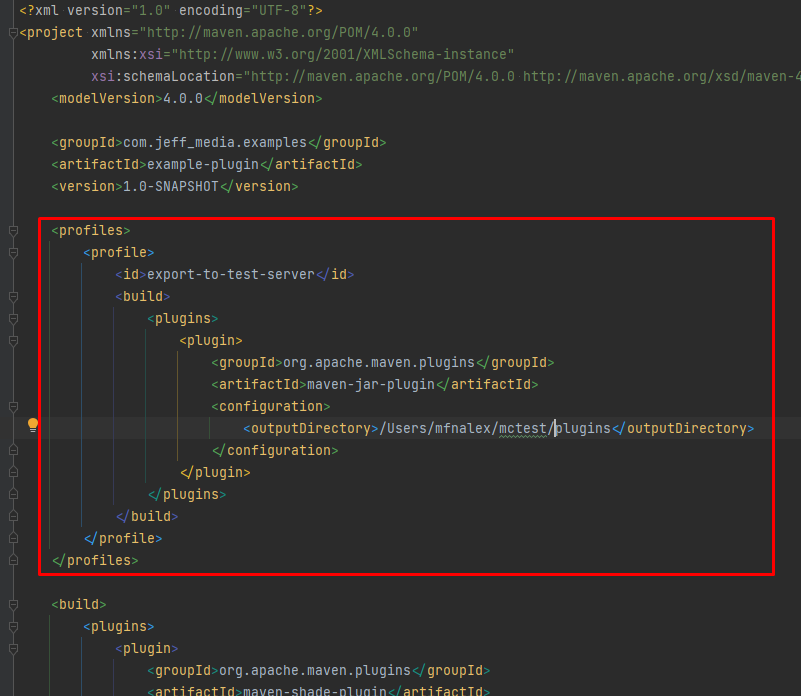
Now, you can decide on every execution whether maven should use the normal path, or your test server’s path, either by declaring it on command line, or from within your IDE:
In IntelliJ
In IntelliJ, you can just enable your profile in the “maven” tab before running “package” or whichever you wanna run:
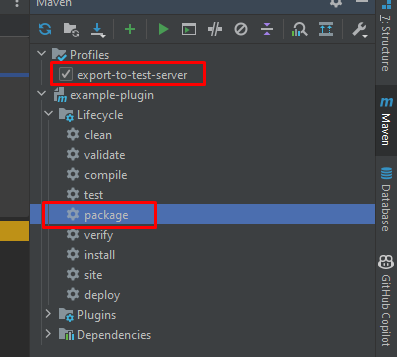
Please note that the profile will of course only show up after you reloaded your pom.xml changes.
From command line
From command line, you can just provide the list of profiles (separated by comma, if you got more than one) by using the -P
argument, like this:
mvn -P export-to-test-server package
3. The wrong way (don’t do this):
Some people also use the global <build><outputDirectory> option, the maven-shade-plugin, the maven-compiler-plugin, or even worse – the specialsource-plugin to change the output directory.
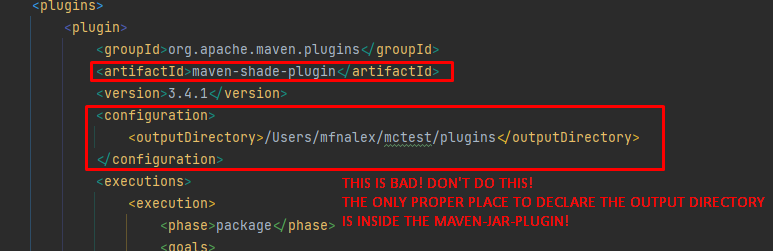
All of these methods are wrong, as they fuck up other lifecycles and/or will result in more than one .jar being saved to your plugins folder.
The only proper way is to declare the output directory in the maven-jar-plugin, preferrably using a profile instead of the global config (as explained above).
What if I have an external server and want to achieve this same affect!
There are multiple ways to do this. One way to do it is using (shameless self-plug) my plugin JenkinsArtifactDownloader. You would set up a jenkins build on git push, put the settings for your jenkins server in the plugin config, and then you could just restart the server whenever you git push and get the changes automatically.
https://www.spigotmc.org/resources/jenkinsartifactdownloader.109014/
A quick and dirty workaround would be to just use the exec-maven-plugin from org.codehaus.mojo, and then let it run scp or similar to put the file whereever you like. For example, in your exec-maven-plugin’s config:
<executable>scp</executable>
<arguments>
<argument>target/MyPlugin-${project.version}.jar</argument>
<argument>myserver:/path/to/test/server/plugins/MyPlugin.jar</argument>
</arguments>